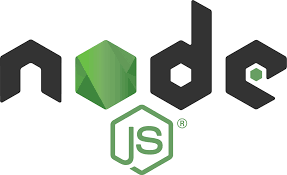
Node.js is an open-source, cross-platform JavaScript runtime environment that allows developers to run JavaScript code server-side. It is built on the V8 JavaScript runtime engine, the same engine that powers the Google Chrome browser. Node.js enables developers to use JavaScript for both server-side and client-side development, offering a unified language for full-stack development.
Key features of Node.js include:
Asynchronous and Non-blocking: Node.js is designed to be non-blocking and asynchronous, allowing it to handle many simultaneous connections without waiting for one to complete before moving on to the next. This makes it well-suited for handling real-time applications, such as chat applications or online gaming.
Event-Driven: Node.js follows an event-driven architecture, using events to manage and handle asynchronous operations. This is facilitated by the EventEmitter module, which allows certain objects (called emitters) to emit named events that can be listened to by functions (listeners) that are registered with those emitters.
NPM (Node Package Manager): Node.js comes with npm, a package manager that allows developers to easily install, share, and manage third-party libraries and tools.
Cross-Platform: Node.js is designed to be cross-platform and runs on various operating systems, including Windows, macOS, and Linux.
Single-Threaded: While Node.js is single-threaded, it uses an event-driven, non-blocking model to handle concurrency. It achieves this through the event loop, allowing it to efficiently handle multiple connections simultaneously.
To get started with Node.js, you typically create a JavaScript file (e.g., app.js) and use the Node.js runtime to execute it. You can handle HTTP requests, create servers, and perform various tasks using the rich set of modules available in the Node.js ecosystem.
Here's a simple example of a basic HTTP server using Node.js:
javascript
// Load the HTTP module to create an HTTP server.
const http = require('http');
// Configure HTTP server to respond with "Hello, World!" to all requests.
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!\n');
});
// Listen on port 3000 and IP address 127.0.0.1.
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
To run this script, save it to a file (e.g., app.js) and execute it using the command node app.js in the terminal.
This is just a basic introduction to Node.js, and there is much more to explore, including the vast ecosystem of npm packages that can be used to enhance and extend Node.js applications.
What is it: Express.js is a web application framework for Node.js. It simplifies the process of building robust web applications and APIs.
Key Features:
Routing: Helps define the structure of your web application or API.
Middleware: Allows you to extend the request and response objects.
Template Engines: Supports various template engines like EJS, Pug, and Handlebars.
Example:
javascript
const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, Express!'); }); app.listen(3000, () => { console.log('Express server listening on port 3000'); });
2. NPM (Node Package Manager):
What is it: npm is the package manager for Node.js. It helps you discover and install packages (libraries and tools) that you can use in your Node.js projects.
Common Commands:
npm install <package>: Installs a package locally.
npm install -g <package>: Installs a package globally.
npm init: Initializes a new package.json file for your project.
npm start: Runs the script specified as the "start" script in your package.json.
Example:
bash
npm install express
3. File System Module:
What is it: The fs module provides an API for interacting with the file system.
Common Operations:
Reading and writing files.
Creating and deleting directories.
File stats and information.
Example:
javascript
const fs = require('fs'); // Reading a file fs.readFile('example.txt', 'utf8', (err, data) => { if (err) throw err; console.log(data); }); // Writing to a file fs.writeFile('example.txt', 'Hello, Node.js!', (err) => { if (err) throw err; console.log('File written successfully.'); });
4. Event Emitters:
What are they: Node.js uses the EventEmitter class to handle events. Many built-in modules inherit from EventEmitter, and you can create your own custom event emitters.
Example:
javascript
const EventEmitter = require('events'); class MyEmitter extends EventEmitter {} const myEmitter = new MyEmitter(); myEmitter.on('event', () => { console.log('Event emitted!'); }); myEmitter.emit('event');
5. Callbacks and Promises:
Callbacks: Node.js is known for its callback-based asynchronous programming style.
javascript
const fetchData = (callback) => { setTimeout(() => { callback('Data fetched!'); }, 2000); }; fetchData((data) => { console.log(data); });
Promises: With the introduction of promises, you can handle asynchronous operations in a more structured way.
javascript
const fetchDataPromise = () => { return new Promise((resolve) => { setTimeout(() => { resolve('Data fetched with Promise!'); }, 2000); }); }; fetchDataPromise().then((data) => { console.log(data); });
These are just a few aspects of Node.js, and there's much more to explore based on your specific use case, such as handling databases (e.g., MongoDB with Mongoose), authentication, testing, and deploying Node.js applications. If you have specific questions or areas you'd like to explore further, feel free to ask!
0 comments
Be the first to comment!
This post is waiting for your feedback.
Share your thoughts and join the conversation.