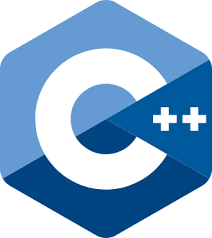
C++ is a general-purpose programming language that was developed as an extension of the C programming language. It was created by Bjarne Stroustrup at Bell Labs in the early 1980s. C++ adds object-oriented programming features to C, which allows for the creation of more modular and reusable code.
Here are some key features and concepts in C++:
Object-Oriented Programming (OOP): C++ supports the four pillars of OOP - encapsulation, inheritance, polymorphism, and abstraction. This enables developers to structure their code in a more organized and modular way.
Classes and Objects: In C++, you can define classes, which are user-defined data types that encapsulate data and the functions that operate on that data. Objects are instances of classes.
Inheritance: This allows a class to inherit properties and behaviors from another class. It promotes code reuse and the creation of a hierarchical class structure.
Polymorphism: C++ supports both compile-time (function overloading) and runtime (virtual functions) polymorphism. Polymorphism allows objects of different types to be treated as objects of a common base type.
Abstraction: Abstraction allows developers to hide the complex implementation details and show only the essential features of an object.
Templates: C++ supports generic programming through templates. This allows you to write code that works with any data type.
Standard Template Library (STL): The STL provides a collection of template classes and functions that implement many popular and commonly used algorithms and data structures like vectors, lists, queues, and more.
Memory Management: C++ allows manual memory management using new and delete operators, but it also provides automatic memory management through smart pointers and containers.
Operators: C++ supports a wide range of operators, both arithmetic and logical, and allows overloading of operators for user-defined types.
Multi-paradigm Language: C++ supports procedural, object-oriented, and generic programming paradigms.
Here's a simple example of a C++ program:
cpp
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program prints "Hello, World!" to the console. The #include directive is used to include the necessary header file for input and output operations (iostream). std::cout is used to print to the console, and std::endl represents the end of a line.
C++ allows the use of pointers, which are variables that store memory addresses. You can manipulate data indirectly through pointers.
cpp
int x = 10; int* ptr = &x; // Pointer to int *ptr = 20; // Changes the value of x through the pointer
References provide an alternative way to alias variables.
cpp
int y = 30; int& ref = y; // Reference to int ref = 40; // Changes the value of y through the reference
File I/O:
C++ supports file input and output operations through streams.
cpp
#include <fstream> #include <iostream> int main() { std::ofstream outfile("example.txt"); outfile << "Hello, File!" << std::endl; outfile.close(); std::ifstream infile("example.txt"); std::string line; while (std::getline(infile, line)) { std::cout << line << std::endl; } infile.close(); return 0; }
Exception Handling:
C++ supports exception handling to deal with runtime errors.
cpp
try { // code that might throw an exception throw std::runtime_error("An error occurred"); } catch (const std::exception& e) { std::cerr << "Exception caught: " << e.what() << std::endl; }
Standard Template Library (STL) Containers:
C++ provides various containers in the STL, such as vectors, lists, maps, queues, and more.
cpp
#include <vector> #include <iostream> int main() { std::vector<int> numbers = {1, 2, 3, 4, 5}; for (const auto& num : numbers) { std::cout << num << " "; } std::cout << std::endl; return 0; }
Lambda Expressions:
C++ supports lambda expressions, which are anonymous functions.
cpp
#include <iostream> int main() { auto add = [](int a, int b) -> int { return a + b; }; std::cout << add(3, 4) << std::endl; // Output: 7 return 0; }
Threading:
C++11 and later versions support multi-threading.
cpp
#include <iostream> #include <thread> void threadFunction() { std::cout << "Hello from thread!" << std::endl; } int main() { std::thread t(threadFunction); t.join(); // Wait for the thread to finish return 0; }
These are just a few additional aspects of C++. The language is vast and versatile, allowing developers to create a wide range of applications, from system-level programming to high-level software development. If you have any specific questions or topics you'd like to explore further, feel free to ask!
0 comments
Be the first to comment!
This post is waiting for your feedback.
Share your thoughts and join the conversation.