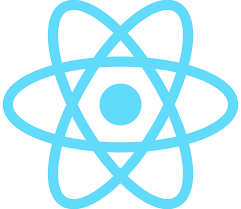
React is a popular JavaScript library for building user interfaces. Developed and maintained by Facebook, React is widely used for creating dynamic and interactive web applications. It follows a component-based architecture, allowing developers to build UIs by creating reusable and modular components.
Here are some key concepts and features of React:
Components: React applications are built using components, which are self-contained, reusable pieces of code that define how a part of the user interface should appear and behave. Components can be nested within each other to create complex UI structures.
JSX (JavaScript XML): React uses JSX, a syntax extension for JavaScript, to describe what the UI should look like. JSX allows developers to write HTML-like code within JavaScript, making it easier to visualize and work with the UI components.
jsx
const element = <h1>Hello, React!</h1>;
Virtual DOM: React uses a virtual DOM to improve performance. Instead of updating the actual DOM directly, React creates a virtual representation of the DOM in memory. When changes occur, React updates the virtual DOM first and then calculates the most efficient way to update the actual DOM, minimizing the number of manipulations needed.
Unidirectional Data Flow: React follows a unidirectional data flow, meaning that data flows in a single direction through the components. This helps maintain a predictable state and makes it easier to understand how data changes affect the UI.
State and Props: React components can have state, which represents the data that can change over time. Props (short for properties) are inputs to a React component that are passed from its parent component. By managing state and props, React allows developers to create dynamic and interactive user interfaces.
Lifecycle Methods: React components have lifecycle methods that allow developers to execute code at specific points in the component's lifecycle, such as when it is first created, updated, or destroyed. This provides control over the rendering process and allows for actions like fetching data or cleaning up resources.
React Hooks: Introduced in React 16.8, hooks are functions that enable developers to use state and other React features in functional components, allowing them to write more concise and readable code.
Some of the commonly used hooks include useState, useEffect, useContext, and useReducer.
Here's a simple example of a functional component using hooks:
jsx
import React, { useState, useEffect } from 'react';
function ExampleComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
React is widely used in the development of single-page applications, and it has a large and active community with a rich ecosystem of libraries and tools. To get started with React, you can use tools like Create React App for setting up a new project quickly. Additionally, React has extensive documentation available on the official website: React Documentation.
Conditional Rendering: React allows for conditional rendering of components, meaning that you can show or hide components based on certain conditions. This is often done using JavaScript expressions within JSX.
jsx
function ConditionalRenderComponent({ isLoggedIn }) { return ( <div> {isLoggedIn ? <p>Welcome, User!</p> : <p>Please log in</p>} </div> ); }
Forms in React: Handling forms in React involves using state to manage form input and handling form submissions. React provides controlled components, where the form elements are controlled by React state.
jsx
import React, { useState } from 'react'; function MyForm() { const [inputValue, setInputValue] = useState(''); const handleChange = (event) => { setInputValue(event.target.value); }; const handleSubmit = (event) => { event.preventDefault(); console.log('Submitted:', inputValue); }; return ( <form onSubmit={handleSubmit}> <input type="text" value={inputValue} onChange={handleChange} /> <button type="submit">Submit</button> </form> ); }
Routing in React: For building single-page applications, routing is essential. React Router is a widely used library for handling navigation and routing in React applications.
jsx
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
function App() {
return (
<Router>
<Switch>
<Route path="/home" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Router>
);
}
Context API: React provides the Context API for managing global state that needs to be accessed by multiple components without having to pass props through each level of the component tree.
jsx
const MyContext = React.createContext();
function MyProvider({ children }) {
const [value, setValue] = useState('Default Value');
return (
<MyContext.Provider value={{ value, setValue }}>
{children}
</MyContext.Provider>
);
}
function MyComponent() {
const { value, setValue } = useContext(MyContext);
return (
<div>
<p>Value: {value}</p>
<button onClick={() => setValue('New Value')}>Change Value</button>
</div>
);
}
Error Boundaries: React 16 introduced the concept of error boundaries, which are components that catch JavaScript errors anywhere in their child component tree and log those errors, display a fallback UI, and prevent the entire component tree from unmounting.
jsx
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
componentDidCatch(error, errorInfo) {
this.setState({ hasError: true });
logErrorToMyService(error, errorInfo);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
These are just a few additional aspects of React. The library is constantly evolving, and the React community continues to contribute to its growth. As you continue to explore React, you may also encounter topics like React Hooks, testing in React, server-side rendering, and more. The official React documentation is an excellent resource for in-depth learning and reference: React Documentation.
0 件のコメント
この投稿にコメントしよう!
この投稿にはまだコメントがありません。
ぜひあなたの声を聞かせてください。